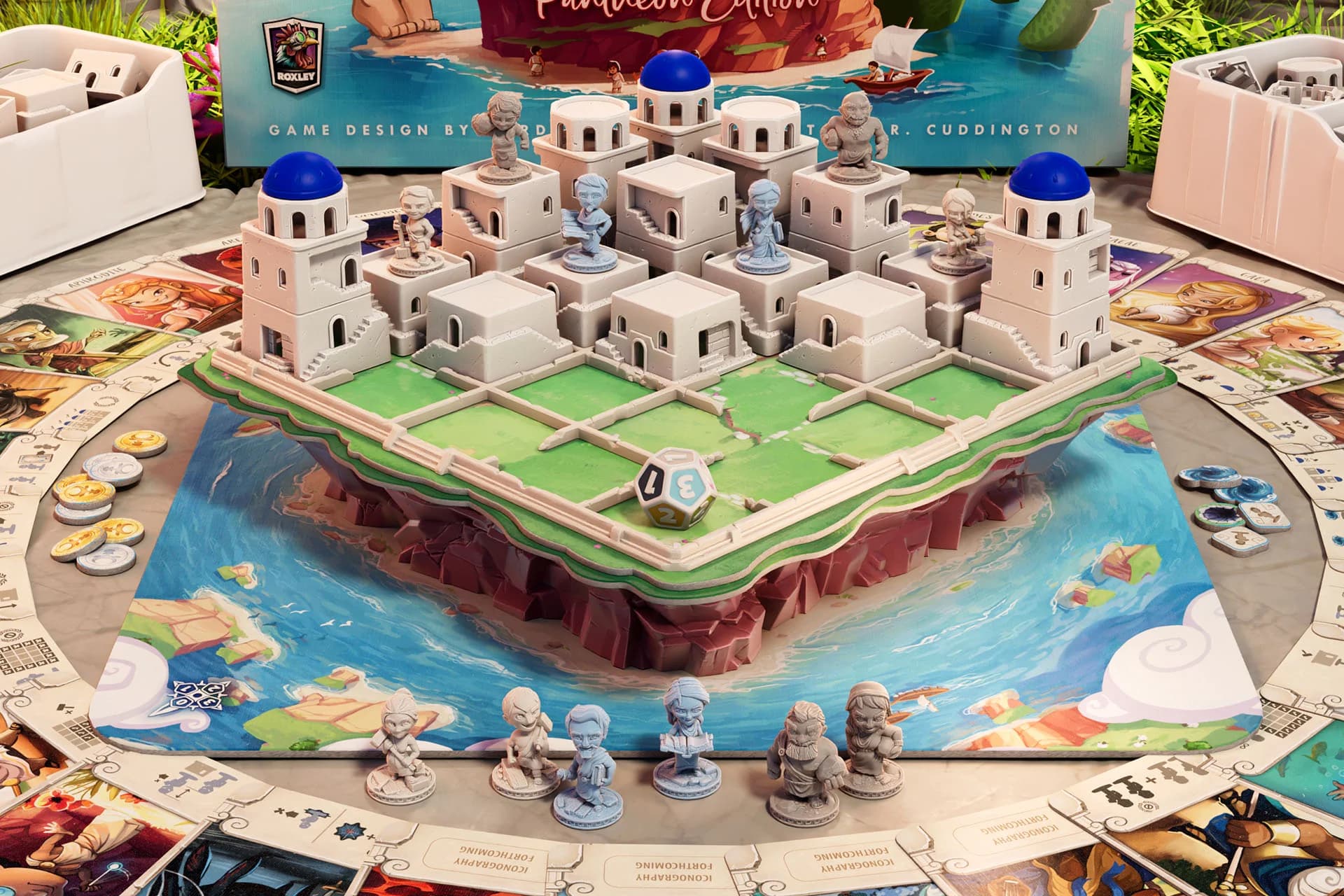
This is a course project of CMU 17-514: Principles of Software Construction. The project is implemented in Java and React (TypeScript).
As per the school's integrity policy, I am not allowed to share any related code publicly. This post serves as a documentation of my design choices and meaningful learnings from the project. With hindsight, I discussed some of the designs that I would have done differently if I were to start over again.
Many thanks to Prof. Claire and Prof. Bogdan for the decent lectures.
Introduction
Santorini is a highly accessible pure-strategy game where you play as a youthful Greek God or Goddess competing to best aid the island's citizens in building a beautiful village in the middle of the Aegean sea. - Roxley
Santorini's basic rule is simple: each player has two workers, and the goal is to build a tower of three floors on a vacant square or move one of your workers onto the third floor. But all sorts of interesting gane extensions are added to make the game, as well as the software design & development, more fun and challenging.
The detailed game rules can be found here.
For this project, the goal is not only to port the real-world game to a web-based version, but also emphasize on the pratices and procedures of software construction, as shown in Fig-1. It is a project that's complex enough to require quite a few planning and design. Most importantly, it provides many hook points for me to apply various design patterns and principles that I learned from the course.
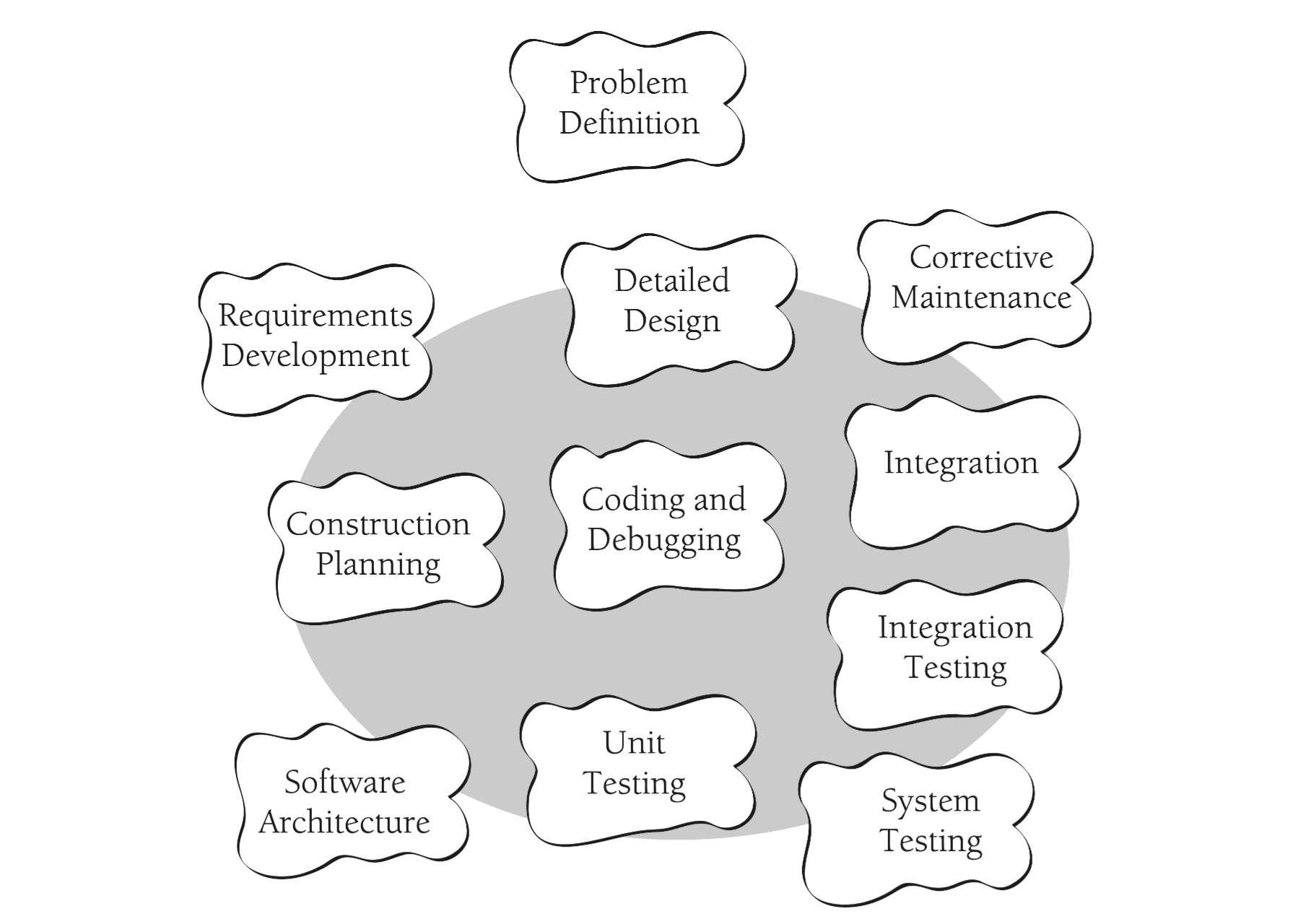
Fig-1 Software Construction Activities. Construction activities are shown inside the gray circle. Construction focuses on coding and debugging but also includes detailed design, unit testing, integration testing, and other activities. - Code Complete 2nd
Detailed Design
This sections shows some key designs of the game, stressing on the first-stage iteration of software construction as elaborated in UML an Patterns. Here are some conciese descriptions of the terminologies:
- Domain Model: A domain model is the most important—and classic—model in object oriented analysis. It illustrates noteworthy concepts in a domain. It can act as a source of inspiration for designing some software objects
- System Sequence Diagram: A system sequence diagram is a picture that shows, for one particular scenario of a use case, the events that external actors generate, their order, and inter-system events. All systems are treated as a black box; the emphasis of the diagram is events that cross the system boundary from actors to systems.

Domain Model
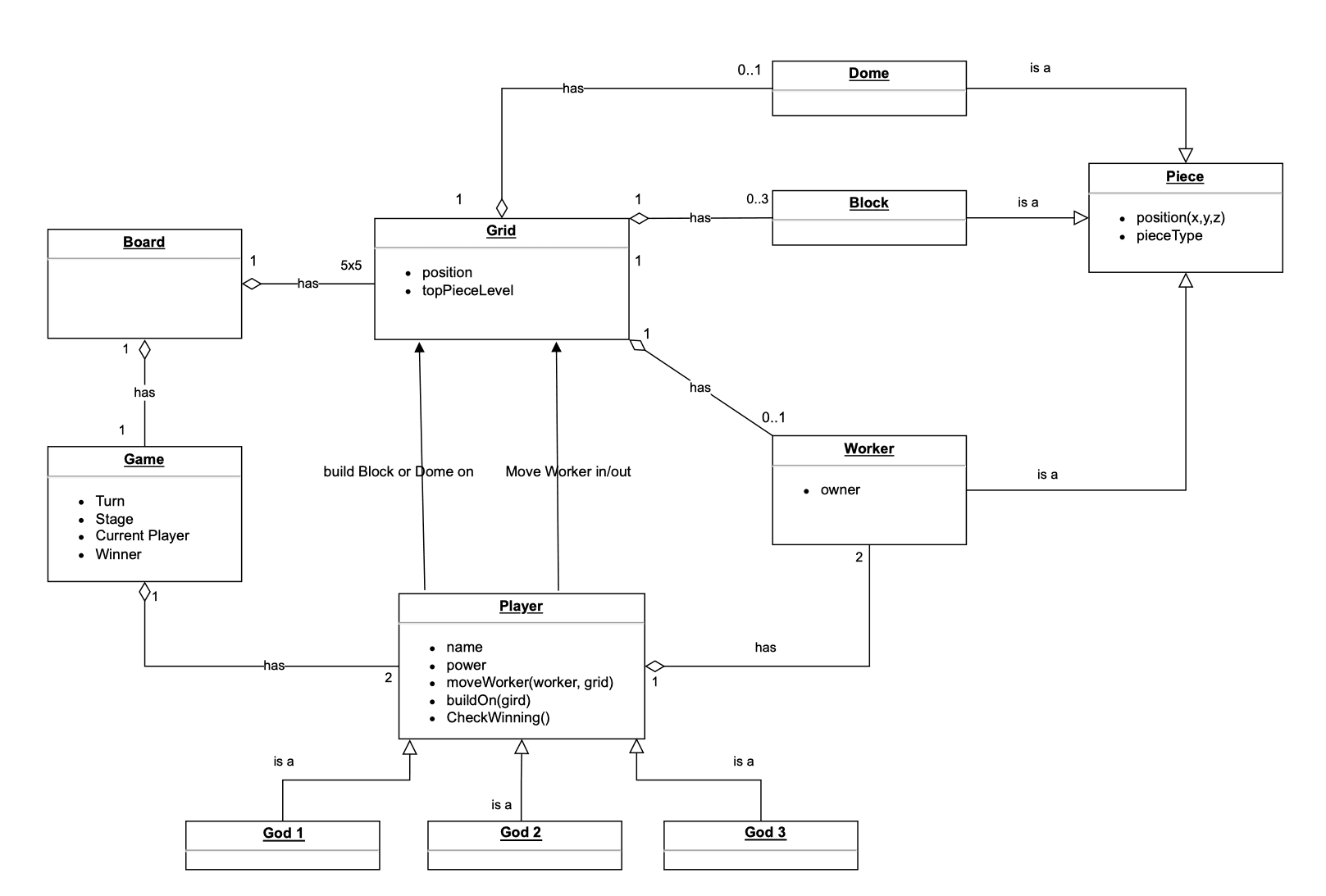
System Sequence Diagram
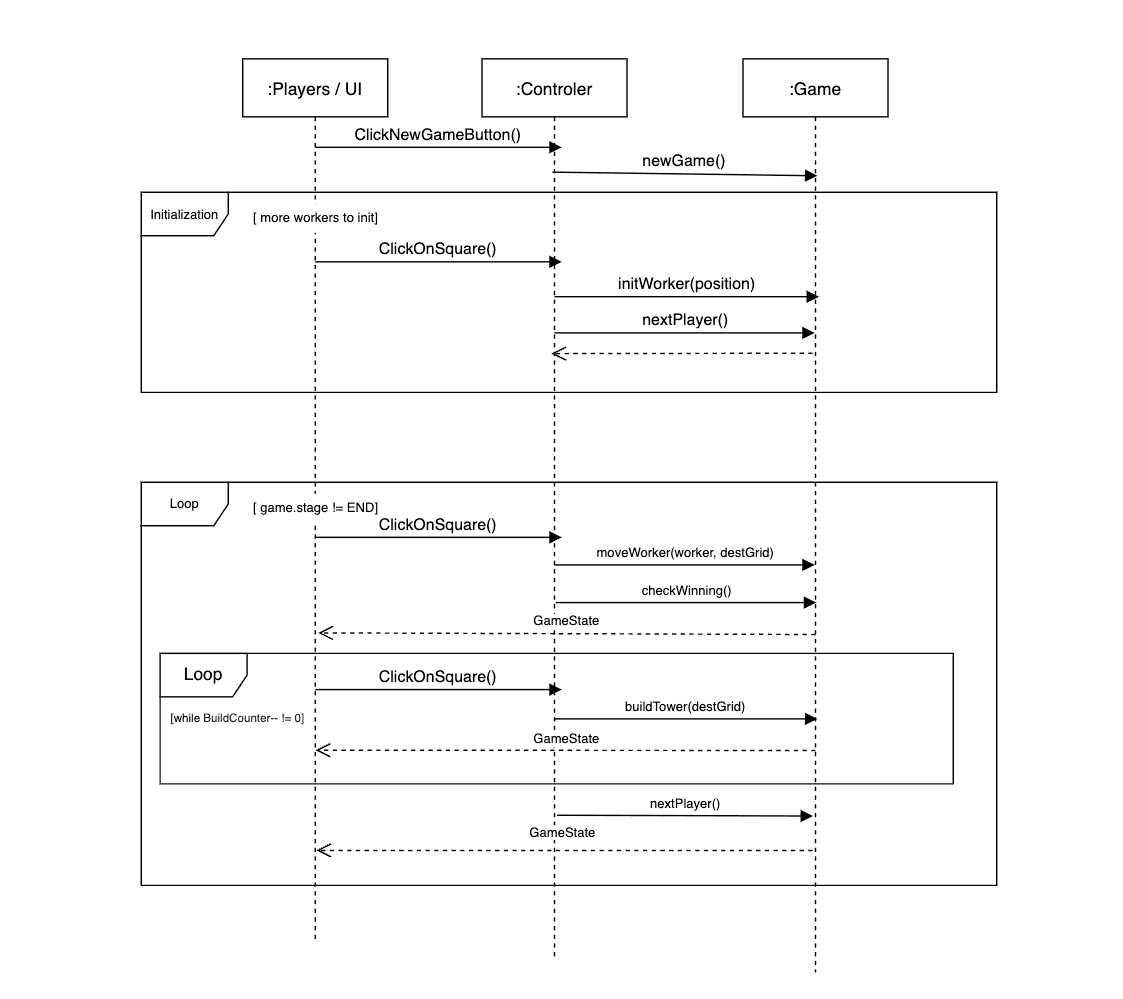
Operation Contracts
This sample contract describes the "move worer" operation in the game. It is a formal description of the operation's preconditions and postconditions. The contract is used to verify the correctness of the operation's implementation.
- Operation: WORKER.moveWorker(Worker worker, Grid dest)
- Preconditions:
- It's the turn of the player who will move the worker.
- The worker is selected by current player.
- The destination for the worker to move into is either empty, or contains a top "Block" that is no higher than the worker by 1 level.
- The destination square must be one of the 8 nearest squares of the worker.
- Postconditions:
- The worker associated with the new square.
- The worker dissociated with the old square.
- The worker's position was updated. The worker's x and y coordinates became the same as those of the new square. And its z coordinate became that of the top piece(would be zero if the new square is empty) plus 1.
- Checked if the current player wins the game. If so, the "Game Stage" became "END", and the game accept no more interactions.
- If the game was not ended, the"Game Stage" changed to "CHOOSE" in which a player is going to choose a square to build.
Implementation
This project is implemented in Java and React (TypeScript). The Java part is the backend server that handles the game logic and state management. The React part is the frontend UI that renders the game and handles user interactions.
Showcase
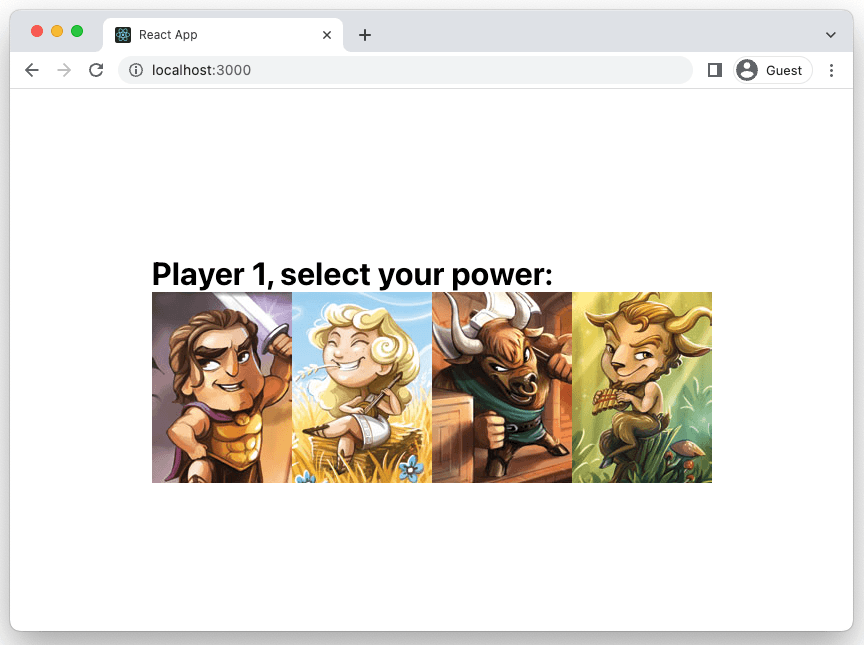
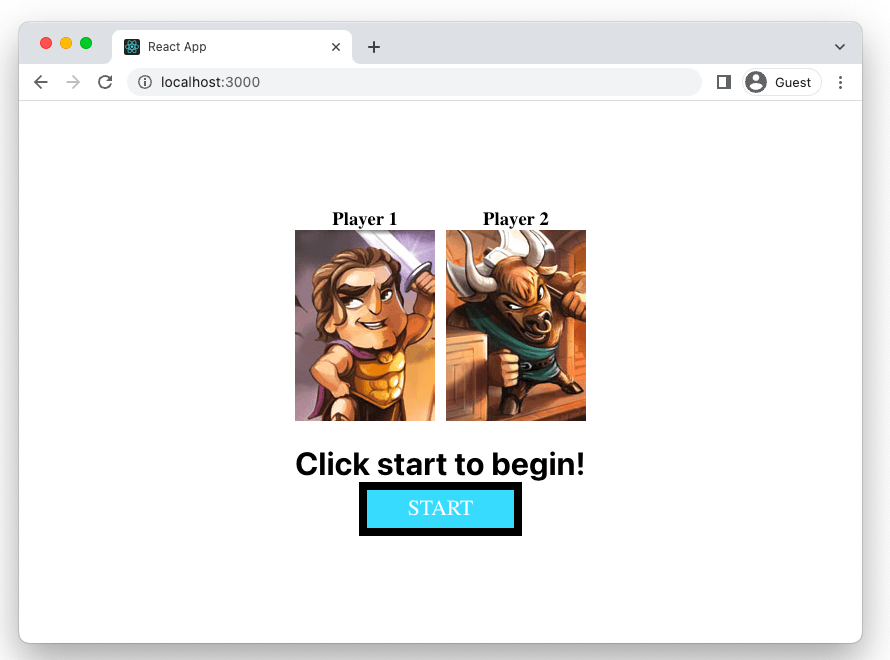
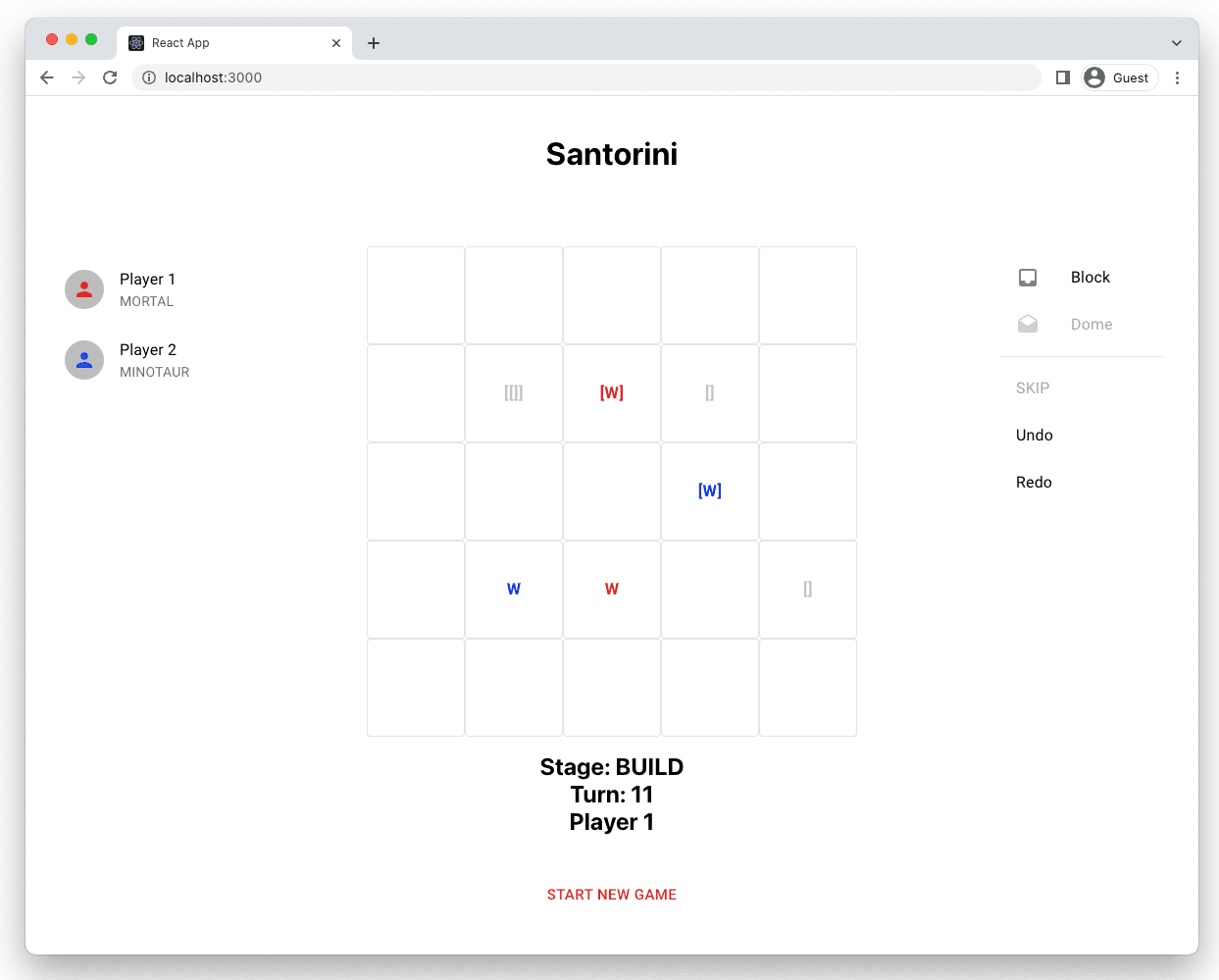
Demo
Live demo is coming soon.